I was working on a feature to migrate Totem to the latest .NETCORE framework upgrade. I was upgrading it to 3.1 version from 2.1. I expected some breaking changes. Microsoft has done a good job of documenting those and providing solutions for those in this MSDN article. Everything went better than expected, and then, the build failed. I looked at the reasons, and it was because of a failing test.
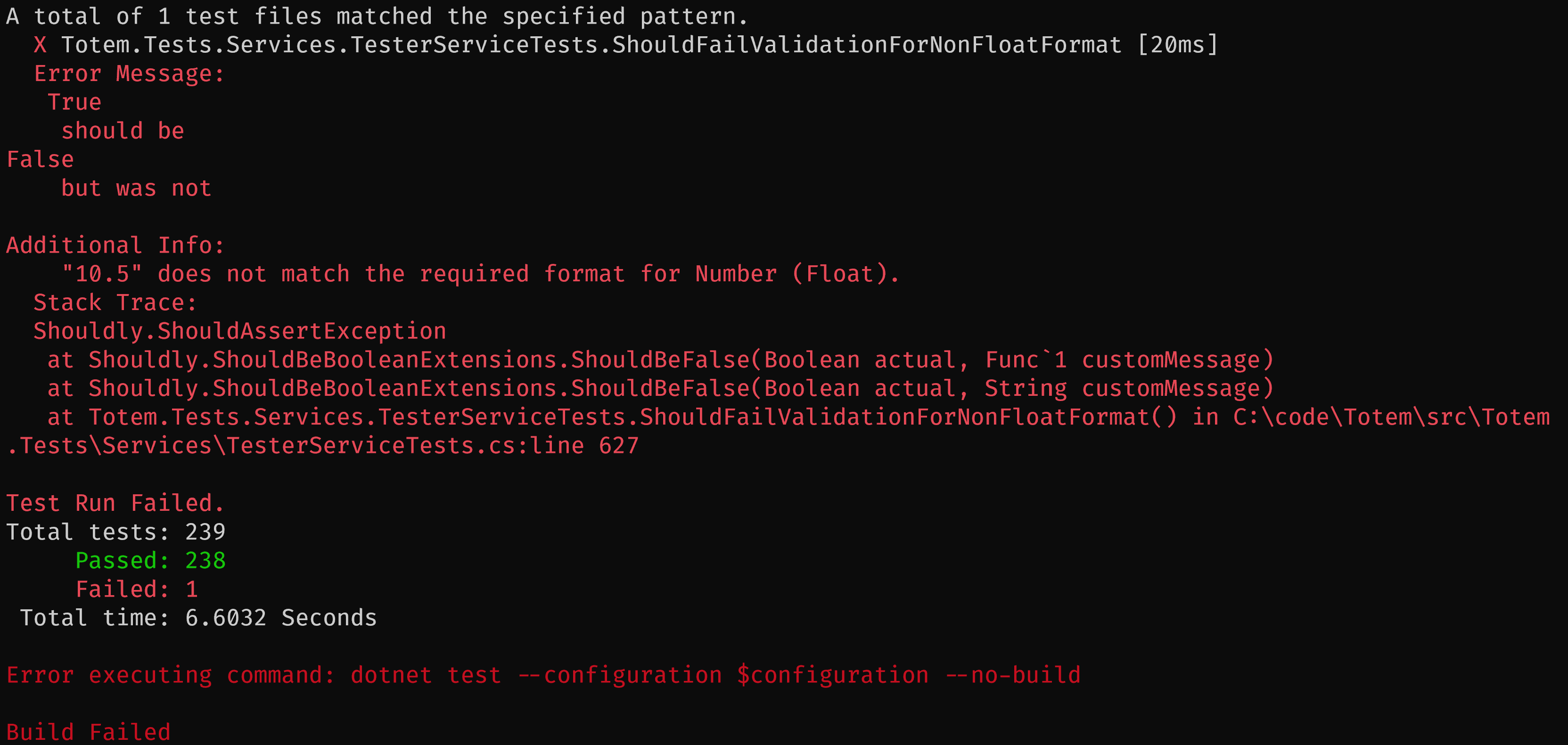
It didn’t make a whole lot of sense. Why would this test fail because of the framework upgrade? I double-checked everything. I then asked my colleague to check running the build on her machine to rule out some environmental silliness. Nope, it broke on her laptop as well. That meant it had to do something in the changes I made.
It came down to lines below:
var isDouble = double.TryParse(kv.Value.ToString(), out _);
var isFloat = float.TryParse(kv.Value.ToString(), out _);
The test was expecting isFloat to be false since it was trying to parse the value of Double.Min. Ok, I didn't touch anything related to these besides the framework update. It must have to do with the framework code, I thought.
After doing a bit of research, I landed on this Github thread. TLDR; The .Parse method doesn’t behave the same way the same as it did in .NETCORE 2.1 and regular .NET framework. It always returns values for formatting purposes. Here is the result of my tests:
.NET Framework 4.7.2
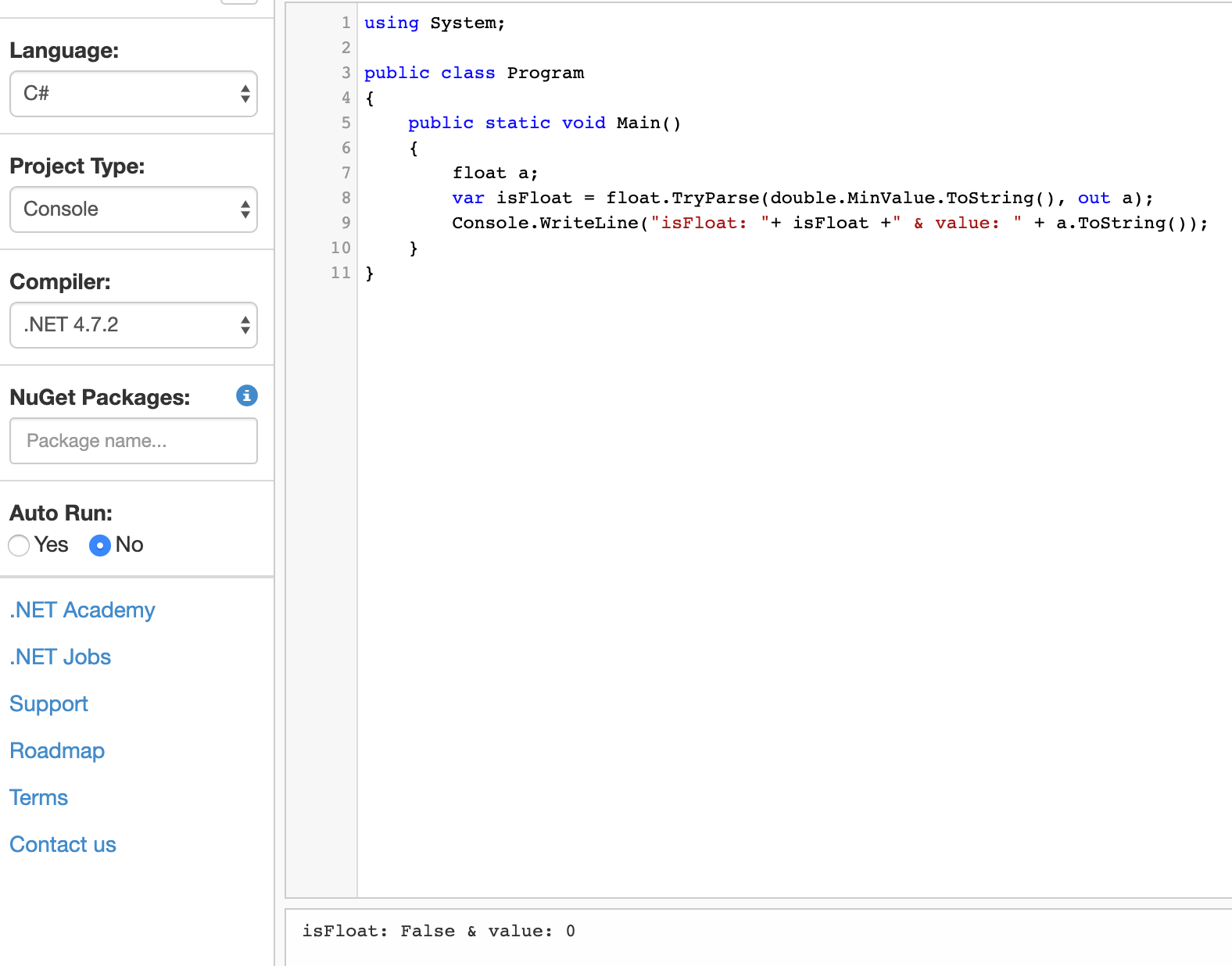
.NETCORE 3.1
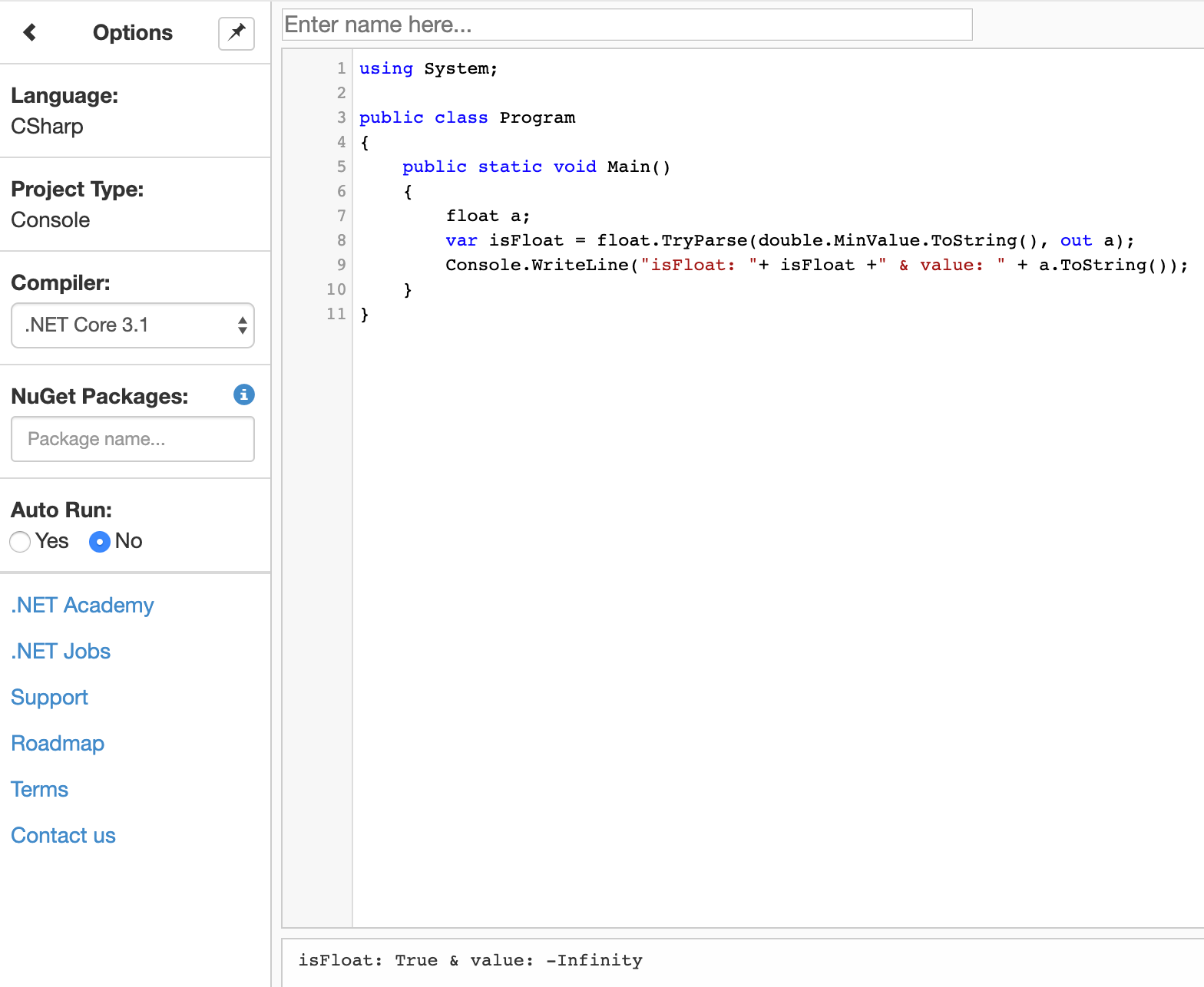
What used to be a False is now a True. What returned 0 is now infinity. I don't know how much code out there is going to break because of this. Anyhow, now, we know what’s wrong, but how do we fix this thing? I landed on this:
var isFloat = float.TryParse(stringVal, out var floatVal)
&& float.IsFinite(floatVal);
For my purpose, I don’t need to do anything special that’s not finite. Checking finiteness was good enough. I added that, and the tests passed.
Is this the best possible fix? At the moment, from my perspective, yes. If I find a better fix, I will post it. Here's the complete pull request to put things in some context.
Comments